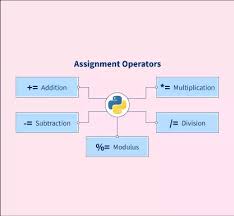
Python is a versatile and powerful programming language, favored by beginners and experts alike for its simplicity and readability. Two essential concepts every Python programmer must understand are assignment operators and exception handling in python. This guide aims to provide a comprehensive introduction to these topics, ensuring you have a solid foundation to build upon as you progress in your Python journey.
Python Assignment Operators
Assignment operators are used to assign values to variables. In Python, the most basic assignment operator is the equal sign =. However, Python provides several other assignment operators that allow you to perform operations and assign values in a single step. These operators not only make the code more concise but also improve its readability.
Basic Assignment Operator
The basic assignment operator = assigns the value on the right to the variable on the left.
python
Copy code
x = 5
y = “Hello, World!”
In the above example, the variable x is assigned the integer value 5, and the variable y is assigned the string value “Hello, World!”.
Compound Assignment Operators
Compound assignment operators combine an arithmetic operation with assignment. These operators include +=, -=, *=, /=, %=, **=, and //=, among others. Let’s explore each of these operators with examples.
Addition Assignment (+=)
The += operator adds the value on the right to the variable on the left and assigns the result to the variable.
python
Copy code
x = 5
x += 3 # Equivalent to x = x + 3
print(x) # Output: 8
Subtraction Assignment (-=)
The -= operator subtracts the value on the right from the variable on the left and assigns the result to the variable.
python
Copy code
x = 5
x -= 2 # Equivalent to x = x – 2
print(x) # Output: 3
Multiplication Assignment (*=)
The *= operator multiplies the variable on the left by the value on the right and assigns the result to the variable.
python
Copy code
x = 5
x *= 2 # Equivalent to x = x * 2
print(x) # Output: 10
Division Assignment (/=)
The /= operator divides the variable on the left by the value on the right and assigns the result to the variable.
python
Copy code
x = 10
x /= 2 # Equivalent to x = x / 2
print(x) # Output: 5.0
Modulus Assignment (%=)
The %= operator performs modulus division on the variable on the left by the value on the right and assigns the result to the variable.
python
Copy code
x = 10
x %= 3 # Equivalent to x = x % 3
print(x) # Output: 1
Exponentiation Assignment (**=)
The **= operator raises the variable on the left to the power of the value on the right and assigns the result to the variable.
python
Copy code
x = 2
x **= 3 # Equivalent to x = x ** 3
print(x) # Output: 8
Floor Division Assignment (//=)
The //= operator performs floor division on the variable on the left by the value on the right and assigns the result to the variable.
python
Copy code
x = 10
x //= 3 # Equivalent to x = x // 3
print(x) # Output: 3
Understanding these Python Assignment Operators is crucial for writing efficient and readable code. They help reduce redundancy and make the code more concise.
Exception Handling in Python
Exception handling is a powerful feature in Python that allows developers to manage and respond to runtime errors gracefully. Without exception handling, any error in your code would cause the program to crash, resulting in a poor user experience. Python provides a robust mechanism to catch and handle exceptions, ensuring your program can recover from errors and continue executing.
The Basics of Exception Handling
In Python, exceptions are handled using the try, except, else, and finally blocks. Here is a basic example:
python
Copy code
try:
# Code that might raise an exception
result = 10 / 0
except ZeroDivisionError:
# Code to handle the exception
print(“Cannot divide by zero!”)
else:
# Code to execute if no exceptions are raised
print(“Division successful!”)
finally:
# Code to execute regardless of whether an exception was raised
print(“Execution complete.”)
The try Block
The try block contains the code that might raise an exception. If an exception occurs within this block, Python immediately stops executing the code in the try block and jumps to the except block.
The except Block
The except block contains the code that handles the exception. You can specify the type of exception you want to catch. In the above example, we catch a ZeroDivisionError.
The else Block
The else block contains code that executes if no exceptions are raised in the try block. This block is optional.
The finally Block
The finally block contains code that executes regardless of whether an exception was raised. This block is also optional but is useful for cleaning up resources, such as closing files or releasing locks.
Handling Multiple Exceptions
You can handle multiple exceptions by specifying multiple except blocks. Each except block can handle a different type of exception.
python
Copy code
try:
# Code that might raise an exception
value = int(input(“Enter a number: “))
result = 10 / value
except ValueError:
# Handle invalid input
print(“Invalid input! Please enter a valid number.”)
except ZeroDivisionError:
# Handle division by zero
print(“Cannot divide by zero!”)
else:
print(“Division successful!”)
finally:
print(“Execution complete.”)
Raising Exceptions
In addition to handling exceptions, you can also raise exceptions using the raise statement. This is useful when you want to trigger an exception based on certain conditions.
python
Copy code
def check_age(age):
if age < 0:
raise ValueError(“Age cannot be negative!”)
else:
print(“Age is valid.”)
try:
check_age(-1)
except ValueError as e:
print(e)
Custom Exceptions
Python allows you to define custom exceptions by creating a new class that inherits from the Exception class. This is useful for creating more specific error messages tailored to your application’s needs.
python
Copy code
class CustomError(Exception):
pass
def check_value(value):
if value > 100:
raise CustomError(“Value cannot exceed 100!”)
else:
print(“Value is within range.”)
try:
check_value(150)
except CustomError as e:
print(e)
Best Practices for Exception Handling
- Catch Specific Exceptions: Always catch specific exceptions rather than using a general except block. This ensures that you handle only the exceptions you expect and avoid masking other issues.
- Use Finally for Cleanup: Use the finally block to release resources, such as file handles or network connections, to avoid resource leaks.
- Avoid Silent Failures: Do not use empty except blocks that silently catch all exceptions. This can make debugging difficult and mask real issues.
- Log Exceptions: Log exceptions to a file or monitoring system to help diagnose issues later.
Understanding and implementing exception handling in Python ensures your programs are robust, user-friendly, and maintainable. By gracefully handling errors, you can prevent your programs from crashing and provide meaningful feedback to users.
Conclusion
Mastering Python Assignment Operators and Exception Handling is fundamental to becoming a proficient Python developer. Assignment operators help make your code concise and readable, while exception handling ensures your programs can handle errors gracefully and continue to run smoothly. By incorporating these concepts into your coding practices, you’ll be well-equipped to tackle more complex programming challenges in Python.