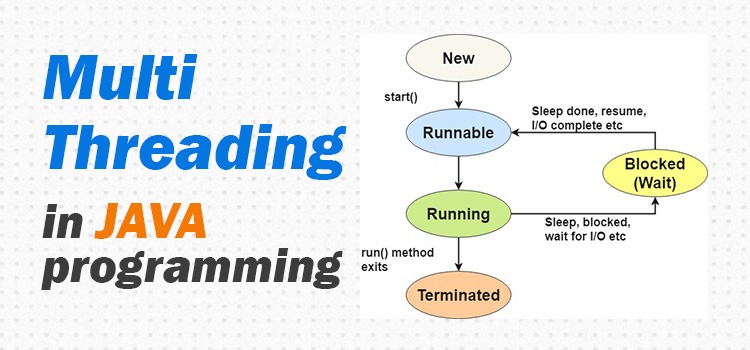
Multithreading is a powerful feature in Java that allows the concurrent execution of two or more parts of a program to maximize the utilization of CPU resources. This Java Multithreading Tutorial will delve into the core concepts, practical applications, and benefits of multithreading in Java. We’ll also cover common Java multithreading interview questions to prepare you for technical interviews.
Understanding Multithreading
Multithreading in Java is a process of executing multiple threads simultaneously. A thread is a lightweight subprocess, the smallest unit of processing. Multithreading is essential for performing complex and resource-intensive tasks without compromising the performance of an application.
Benefits of Multithreading in Java
-
Improved Performance: By executing multiple threads in parallel, Java applications can complete tasks more quickly and efficiently.
-
Resource Sharing: Threads within the same process share the same memory space, which allows for efficient resource utilization.
-
Scalability: Multithreading makes it easier to scale applications to handle increased loads by leveraging multiple cores and processors.
-
Responsive User Interfaces: In GUI applications, multithreading ensures that the user interface remains responsive even when performing long-running tasks.
Key Concepts in Java Multithreading
Thread Class and Runnable Interface
In Java, there are two main ways to create a thread:
Extending the Thread Class: You can create a new thread by extending the Thread class and overriding its run method.
java
Copy code
class MyThread extends Thread {
public void run() {
System.out.println(“Thread is running…”);
}
public static void main(String[] args) {
MyThread thread = new MyThread();
thread.start();
}
}
Implementing the Runnable Interface: You can also create a thread by implementing the Runnable interface and passing an instance of the implementing class to a Thread object.
java
Copy code
class MyRunnable implements Runnable {
public void run() {
System.out.println(“Thread is running…”);
}
public static void main(String[] args) {
MyRunnable myRunnable = new MyRunnable();
Thread thread = new Thread(myRunnable);
thread.start();
}
}
Thread Lifecycle
A thread in Java goes through several stages in its lifecycle:
-
New: The thread is created but not yet started.
-
Runnable: The thread is ready to run and waiting for CPU time.
-
Running: The thread is currently executing.
-
Blocked: The thread is waiting for a resource or condition before it can proceed.
-
Terminated: The thread has finished executing.
Synchronization
When multiple threads access shared resources, there’s a risk of data inconsistency and race conditions. Java provides synchronization mechanisms to ensure that only one thread can access a resource at a time. This is achieved using the synchronized keyword.
java
Copy code
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
public class SyncDemo {
public static void main(String[] args) {
Counter counter = new Counter();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
t1.start();
t2.start();
try {
t1.join();
t2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(“Final count: ” + counter.getCount());
}
}
Common Java Multithreading Interview Questions
To help you prepare for interviews, here are some frequently asked Java multithreading interview questions:
-
What is multithreading in Java? Multithreading in Java is the capability to run multiple threads concurrently within a program, allowing for parallel execution of tasks.
-
How do you create a thread in Java? You can create a thread by extending the Thread class or by implementing the Runnable interface.
-
What is the difference between the start() and run() methods in Java threads? The start() method begins the execution of a new thread, whereas the run() method contains the code to be executed by the thread.
-
What are the states of a thread in its lifecycle? The states are New, Runnable, Running, Blocked, and Terminated.
-
What is synchronization in Java? Synchronization is a mechanism that ensures that only one thread can access a resource at a time to prevent data inconsistency and race conditions.
Advanced Multithreading Topics
Thread Pools
Thread pools manage a pool of worker threads to perform tasks. Using a thread pool can improve performance and resource management in applications. The ExecutorService interface in the java.util.concurrent package provides a way to create and manage thread pools.
java
Copy code
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolDemo {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
executorService.execute(() -> {
System.out.println(Thread.currentThread().getName() + ” is running”);
});
}
executorService.shutdown();
}
}
Callable and Future
The Callable interface is similar to Runnable, but it can return a result and throw a checked exception. The Future interface represents the result of an asynchronous computation.
java
Copy code
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class CallableDemo {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(3);
Callable<Integer> task = () -> {
return 123;
};
Future<Integer> future = executorService.submit(task);
try {
Integer result = future.get();
System.out.println(“Result: ” + result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
executorService.shutdown();
}
}
Conclusion
Multithreading in Java is a fundamental concept that can significantly enhance the performance and responsiveness of applications. Understanding the basics, such as thread creation and synchronization, as well as advanced topics like thread pools and the Callable interface, is essential for any Java developer. By mastering these concepts, you’ll be well-prepared for any Java multithreading interview and capable of building efficient, concurrent Java applications.