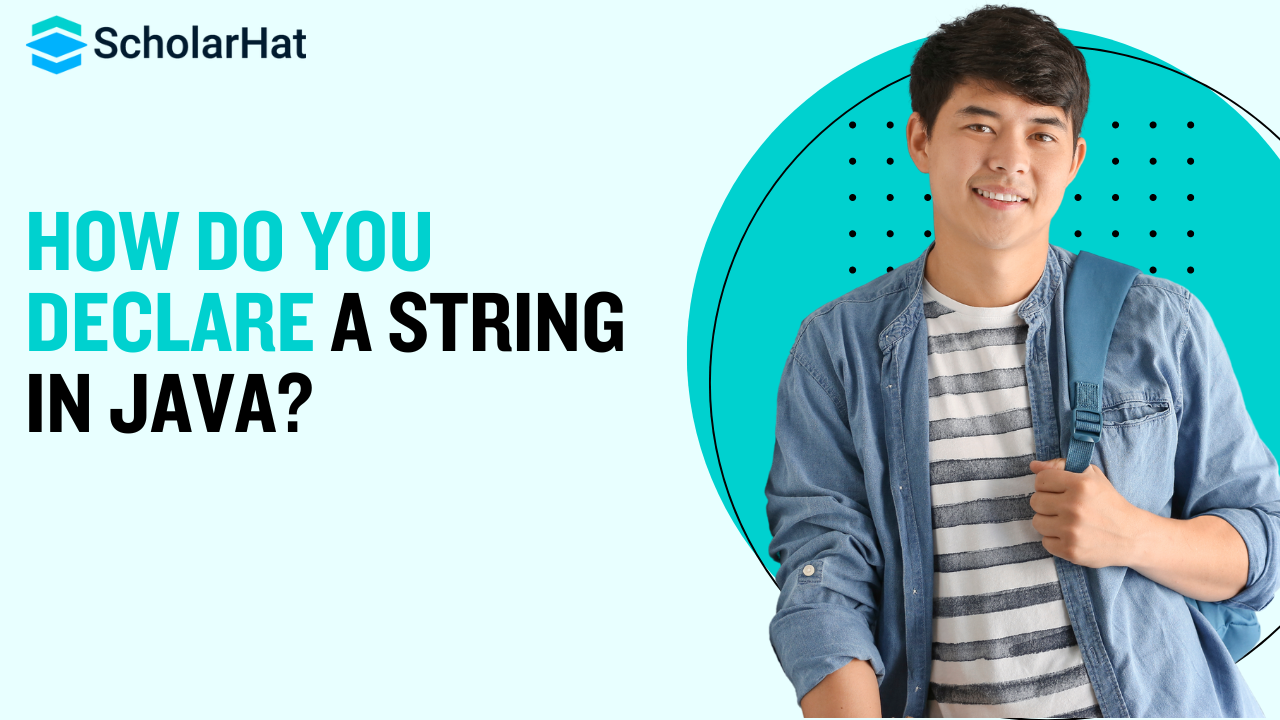
In Java programming, understanding how to handle strings is fundamental. One of the first questions you might encounter is, “How do you declare a string in Java?” In this article, we’ll explore this topic in-depth, covering various methods and best practices to help you master string manipulation in Java.
Introduction to Strings in Java
Strings in Java are a sequence of characters. Unlike in C, where strings are arrays of characters, Java treats strings as objects. This approach offers a multitude of methods and functionality to manipulate and utilize strings efficiently.
For those familiar with working with a string in C, the transition to Java’s string handling will present both similarities and differences. Understanding these nuances is crucial for effective Java programming.
Declaring a String in Java
Basic Declaration
In Java, you can declare a string using the String class. Here’s the simplest way to declare a string:
String message;
This declaration creates a string variable named message, but it doesn’t initialize it.
Initialization
You can declare and initialize a string in a single statement:
String greeting = “Hello, World!”;
Here, greeting is declared as a string and initialized with the value “Hello, World!”.
Using the new Keyword
Strings in Java can also be created using the new keyword, though it’s less common:
String welcome = new String(“Welcome to Java!”);
This method explicitly creates a new String object.
String Concatenation
String concatenation is the process of combining two or more strings. In Java, you can concatenate strings using the + operator or the concat method.
Using the + Operator
String firstName = “John”;
String lastName = “Doe”;
String fullName = firstName + ” ” + lastName;
System.out.println(fullName); // Outputs: John Doe
Using the concat Method
String part1 = “Hello, “;
String part2 = “World!”;
String message = part1.concat(part2);
System.out.println(message); // Outputs: Hello, World!
Common String Methods in Java
Java provides a rich set of methods to manipulate strings. Here are some commonly used methods:
Length of a String
The length method returns the number of characters in a string.
String example = “Hello”;
int length = example.length();
System.out.println(“Length: ” + length); // Outputs: Length: 5
Character at Specific Position
The charAt method returns the character at a specified index.
String example = “Hello”;
char letter = example.charAt(1);
System.out.println(“Character at index 1: ” + letter); // Outputs: Character at index 1: e
Substring Extraction
The substring method extracts a portion of a string.
String example = “Hello, World!”;
String sub = example.substring(7, 12);
System.out.println(“Substring: ” + sub); // Outputs: Substring: World
String Comparison
Java provides multiple methods to compare strings: equals, equalsIgnoreCase, compareTo, and more.
String str1 = “Hello”;
String str2 = “hello”;
boolean isEqual = str1.equals(str2); // false
boolean isEqualIgnoreCase = str1.equalsIgnoreCase(str2); // true
System.out.println(“Equals: ” + isEqual); // Outputs: Equals: false
System.out.println(“Equals Ignore Case: ” + isEqualIgnoreCase); // Outputs: Equals Ignore Case: true
For more detailed information on string methods, you can refer to this comprehensive string in Java tutorial.
Advanced String Operations
String Immutability
In Java, strings are immutable, meaning once a string is created, it cannot be changed. Any modification to a string results in the creation of a new string.
String original = “Immutable”;
String modified = original.replace(“Immutable”, “Mutable”);
System.out.println(“Original: ” + original); // Outputs: Original: Immutable
System.out.println(“Modified: ” + modified); // Outputs: Modified: Mutable
StringBuilder and StringBuffer
For scenarios requiring frequent modifications to strings, StringBuilder and StringBuffer are used. These classes provide mutable string objects.
StringBuilder Example
StringBuilder sb = new StringBuilder(“Hello”);
sb.append(“, World!”);
System.out.println(sb.toString()); // Outputs: Hello, World!
StringBuffer Example
StringBuffer is similar to StringBuilder but is thread-safe, making it suitable for multi-threaded applications.
StringBuffer sb = new StringBuffer(“Hello”);
sb.append(“, World!”);
System.out.println(sb.toString()); // Outputs: Hello, World!
String Formatting
Java offers various ways to format strings, enhancing readability and functionality.
Using String.format
The String.format method provides a way to format strings with placeholders.
String name = “John”;
int age = 25;
String formatted = String.format(“Name: %s, Age: %d”, name, age);
System.out.println(formatted); // Outputs: Name: John, Age: 25
Using printf
The printf method is similar to String.format, but it outputs directly to the console.
String name = “Jane”;
int age = 30;
System.out.printf(“Name: %s, Age: %d%n”, name, age); // Outputs: Name: Jane, Age: 30
Practical Examples of String Usage in Java
User Input Validation
Strings are often used to validate user input in applications.
import java.util.Scanner;
public class UserInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter your name: “);
String name = scanner.nextLine();
if (name.isEmpty()) {
System.out.println(“Name cannot be empty!”);
} else {
System.out.println(“Hello, ” + name);
}
scanner.close();
}
}
Reading and Writing Files
Strings play a crucial role in file operations, such as reading from and writing to files.
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
public class FileOperations {
public static void main(String[] args) {
String path = “example.txt”;
try {
String content = new String(Files.readAllBytes(Paths.get(path)));
System.out.println(“File Content: ” + content);
String newText = “Adding new content!”;
Files.write(Paths.get(path), newText.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Common Mistakes and Best Practices
Avoiding NullPointerException
Always check for null before performing operations on strings to avoid NullPointerException.
String str = null;
if (str != null && str.length() > 0) {
System.out.println(“String is not empty”);
} else {
System.out.println(“String is null or empty”);
}
Using equals Instead of ==
Use equals to compare string values, not ==, which compares object references.
String str1 = “Java”;
String str2 = new String(“Java”);
if (str1.equals(str2)) {
System.out.println(“Strings are equal”);
} else {
System.out.println(“Strings are not equal”);
}
Conclusion
In conclusion, declaring and using a string in Java is a foundational skill that every Java programmer must master. From basic declaration to advanced operations, strings in Java offer a wide array of functionalities that make handling text data efficient and straightforward. By understanding these concepts and best practices, you can write more robust and efficient Java programs.
Whether you are dealing with a string in C or a string in Java, mastering strings is essential for any programmer. Embrace the power of strings in Java, and elevate your coding skills to new heights.